If you’re using the Vue CLI to scaffold you VueJS projects (and if you aren’t, you should be) you’ve surely noticed that when you create a new project it will generate a number of files for you. One of these being main.js.
If you open up main.js you will find something similar to the following:
import Vue from 'vue';
import App from './app/App.vue';
new Vue({
render: h => h(App)
}).$mount('#app');
After having opened and gazed upon said file, you may be wondering to yourself…what the heck is that render thingy…and what about that h?
Lets take this apart piece by piece
On the options object that we’re passing to the Vue constructor function, we have a function called render. Its written above as an ES6 arrow function but we could also write it as:
new Vue({
render: function(h) {
return h(App);
}
}).$mount('#app');
To that render function we are passing the argument: h
h is short-hand for createElement() in HyperScript. HyperScript is a language that creates HTML. (read more about HyperScript here) So, if we expand the example above even more we get:
new Vue({
render: function(createElement) {
return createElement(App);
}
}).$mount('#app');
If you have been working on the web for any period of time, this createElement() method should look very familiar. If you want to review its functionality, check out the MDN docs here.
To the createElement method, we are passing a reference to the App component that we imported at the top of the file.
import App from './app/App.vue';
A .vue file is what is known as a SFC or Single File Component. The browser has no idea what this file is but thats ok because during the build process it is parsed with the vue-loader plugin and inserted into the DOM using the $mount() function exposed on the root Vue instance. You can read more about that here.
Turning our attention back to the render function, in this instance we are passing a Single File Component to it to be rendered but we dont have to do that. What if we just wanted to create a simple DIV and have it inserted into the DOM? We could do something like this:
new Vue({
render(createElement) {
return createElement('div', 'hello from my newly created div');
}
}).$mount("#app");
which would give me:
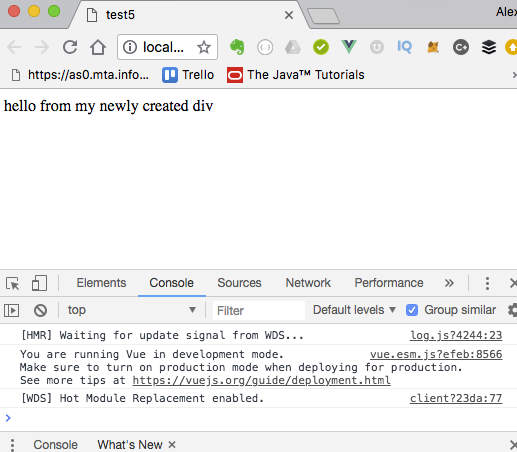
Now, granted, this isnt a real life example of what you can do with the render function but it does show you to power it can provide.
Most of the time, templates will be sufficient for our application development needs but at those times where we may need a little more flexibility and power, it nice to know that we can drop down to the render function and get the full power of JavaScript.
Thanks for reading.